Ein Grafikprogramm
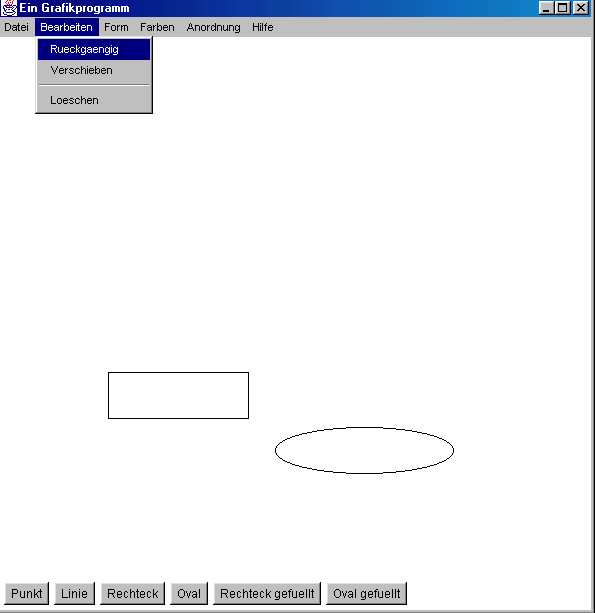
Dies Programm war das Semesterprojelt bei der Veranstaltung Systematisches Programmieren. Die Anforderungen waren: Sechs Zeichenobjekte zu implementieren. Zu diesen Objekten gehörten:
- Punkte,
- Linien,
- Rechtecke und gefüllte Rechtecke,
- Ovale und gefüllte Ovale.
Dies sollte als Applikation mit einer Menüauswahl geschrieben werden. Dieses Menü sollte die Funktionen Löschen, Speichern, Laden, Beenden, Rückgängig und verschiedene Farbauswahlen haben. Mein Programm hat noch die Zusatzfunktionen:
- Verschieben,
- Skalieren,
- Löschen von einzelnen Objekten,
- Dialogfenster beim Speichern und Laden,
- ändern der Farbe
Programmcode
Die Zeichenobjekte
Grafikeindim.java |
---|
1 /** 2 * 3 * Die Zeichenobjekte für das Grafikprogramm 4 * 5 * @version 1.0 vom 27.05.02 6 * @ Xenia Rendtel 7 */ 8 9 import java.awt.*; 10 import java.awt.event.*; 11 import java.util.*; 12 import javax.swing.event.*; 13 import java.io.*; 14 import java.lang.*; 15 16 17 18 abstract class Grafikeindim implements Serializable{ 19 20 protected int x1, y1, x2, y2; 21 protected Color farbe; // die Variablem 22 protected Color ffarbe; 23 protected boolean ausgewaehlt = false; 24 25 26 public void SetzePos(int x1, int y1) { // Anfangsposition setzen 27 this.x1 = x1; 28 this.y1 = y1; 29 30 } 31 32 public void SetzePosrel(int x, int y) { //anfang auf x,y, Verhältnisse aber beibehalten 33 this.x2 = this.x2-this.x1+x; 34 this.y2 = this.y2-this.y1+y; 35 this.x1 = x; 36 this.y1 = y; 37 } 38 39 public void SetzePositionen(int x1, int y1, int x2, int y2) { 40 this.x1 = Math.min(x1, x2); 41 this.y1 = Math.min(y1, y2); 42 this.x2 = Math.max(x1, x2); 43 this.y2 = Math.max(y1, y2); 44 } 45 46 47 // die einzelnen Positionen 48 public int LeseX1() { 49 return x1; 50 } 51 52 public int LeseY1() { 53 return y1; 54 } 55 56 public int LeseX2() { 57 return x2; 58 } 59 60 public int LeseY2() { 61 return y2; 62 } 63 64 65 66 // Farben werden gesetzt 67 public void SetzeFarbe(Color farbe) { 68 this.farbe = farbe; 69 } 70 71 public Color LeseFarbe() { 72 return this.farbe; 73 } 74 75 76 77 public abstract void Zeichne(Graphics g); 78 public abstract boolean contains(Point p); 79 } 80 81 82 class Punkt extends Grafikeindim { 83 84 /* in der Zeichne Methode ist auch das zeichnen enthalten, 85 was man nutzt, wenn man etwas auswählt */ 86 public void Zeichne(Graphics g) { // Ein Punkt ist eine Linie ohne Ausdehnung 87 g.setColor(farbe); 88 g.drawLine(x1, y1, x1, y1); 89 if (ausgewaehlt == true) { 90 g.setColor(new Color(255,0,255)); 91 g.drawRect(x1-3, y1-3, 6, 6); // etwas größer, damit man was sieht 92 } 93 } 94 95 96 97 public boolean contains(Point p) { 98 return (new Rectangle(x1-1, y1-1, 3, 3).contains(p)); 99 } 100 } 101 102 103 104 105 abstract class Grafikzweidim extends Grafikeindim { 106 107 protected Color ffarbe; 108 109 110 111 public void SetzePositionen(int x1, int y1, int x2, int y2) { 112 this.x1 = Math.min(x1, x2); 113 this.y1 = Math.min(y1, y2); 114 this.x2 = Math.max(x1, x2); 115 this.y2 = Math.max(y1, y2); 116 } 117 118 119 120 121 122 public void Zeichne(Graphics g) { 123 if (ausgewaehlt == true) { 124 g.setColor(new Color(255,0,255)); 125 g.drawRect(Math.min(x1, x2), Math.min(y1, y2), Math.abs(x1 - x2), 126 Math.abs(y1 - y2)); 127 } 128 } 129 130 public boolean contains(Point p) { 131 return (new Rectangle(Math.min(x1, x2), Math.min(y1, y2), Math.abs(x1 - x2), 132 Math.abs(y1 - y2)).contains(p)); 133 } 134 135 136 } 137 138 interface Voll { 139 public abstract void SetzeFuellfarbe(Color ffarbe); 140 public abstract Color LeseFuellfarbe(); 141 } 142 143 144 145 146 class Strecke extends Grafikzweidim { 147 148 public void Zeichne(Graphics g) { 149 if (ausgewaehlt == true) { 150 g.setColor(new Color(255,0,255)); 151 g.drawLine(x1+2, y1, x2 +2, y2); 152 g.drawLine(x1-2, y1, x2 -2, y2); 153 g.drawLine(x1-2, y1, x1 +2, y1); 154 g.drawLine(x2-2, y2, x2 +2, y2); 155 } 156 g.setColor(farbe); 157 g.drawLine(x1, y1, x2, y2); 158 } 159 160 161 public boolean contains(Point p) { 162 return (new Rectangle(Math.min(x1, x2), Math.min(y1, y2), Math.abs(x1 - x2), 163 Math.abs(y1 - y2)).contains(p)); 164 } 165 public void SetzePositionen(int x1, int y1, int x2, int y2) { 166 this.x1 = x1; 167 this.y1 = y1; 168 this.x2 = x2; 169 this.y2 = y2; 170 } 171 } 172 173 174 class Rechteck extends Grafikzweidim { 175 176 /* es werden hier die Anfangs und Endpositionen angegeben und nicht 177 Höhe und Breite, da man dies später dann als die Mauspositionen 178 übergeben kann 179 Es gibt hier wie bei Störe meine Kreisenicht vier Fälle, wo die zweite Position 180 liegen kann, diese werden im folgenden abgearbeitet. 181 */ 182 183 public void Zeichne(Graphics g) { 184 if (ausgewaehlt == true) { 185 g.setColor(new Color(255,0,255)); 186 g.drawRect(x1-2, y1-2, x2 - x1+4, y2 - y1+4); 187 } 188 g.setColor(farbe); 189 g.drawRect(Math.min(x1, x2), Math.min(y1, y2), Math.abs(x1 - x2), 190 Math.abs(y1 - y2)); 191 } 192 193 194 195 196 } 197 198 199 200 class Rechteckgef extends Rechteck implements Voll { 201 202 public void Zeichne(Graphics g) { 203 if (ausgewaehlt == true) { 204 g.setColor(new Color(255,0,255)); 205 g.drawRect(x1-2, y1-2, x2 - x1+4, y2 - y1+4); 206 } 207 g.setColor(ffarbe); 208 g.fillRect(Math.min(x1, x2), Math.min(y1, y2), Math.abs(x1 - x2), 209 Math.abs(y1 - y2)); 210 g.setColor(farbe); 211 g.drawRect(Math.min(x1, x2), Math.min(y1, y2), Math.abs(x1 - x2), 212 Math.abs(y1 - y2)); 213 214 } 215 216 217 //Setzen der Füllfarbe 218 public void SetzeFuellfarbe(Color ffarbe) { 219 this.ffarbe = ffarbe; 220 } 221 222 public Color LeseFuellfarbe() { 223 return this.ffarbe; 224 } 225 226 } 227 228 229 class Oval extends Grafikzweidim { 230 231 public void Zeichne(Graphics g) { 232 if (ausgewaehlt == true) { 233 g.setColor(new Color(255,0,255)); 234 g.drawOval(x1-2, y1-2, x2 - x1+4, y2 - y1+4); 235 } 236 g.setColor(farbe); 237 g.drawOval(Math.min(x1, x2), Math.min(y1, y2), Math.abs(x1 - x2), 238 Math.abs(y1 - y2)); 239 240 } 241 242 243 244 } 245 246 247 class Ovalgef extends Oval implements Voll { 248 249 public void Zeichne(Graphics g) { 250 if (ausgewaehlt == true) { 251 g.setColor(new Color(255,0,255)); 252 g.drawOval(x1-2, y1-2, x2 - x1+4, y2 - y1+4); 253 } 254 g.setColor(ffarbe); 255 g.fillOval(Math.min(x1, x2), Math.min(y1, y2), Math.abs(x1 - x2), 256 Math.abs(y1 - y2)); 257 g.setColor(farbe); 258 g.drawOval(Math.min(x1, x2), Math.min(y1, y2), Math.abs(x1 - x2), 259 Math.abs(y1 - y2)); 260 261 } 262 263 //Setzen der Füllfarbe 264 public void SetzeFuellfarbe(Color ffarbe) { 265 this.ffarbe = ffarbe; 266 } 267 268 269 public Color LeseFuellfarbe() { 270 return this.ffarbe; 271 } 272 273 274 public boolean contains(Point p) { 275 return (new Rectangle(Math.min(x1, x2), Math.min(y1, y2), Math.abs(x1 - x2), 276 Math.abs(y1 - y2)).contains(p)); 277 } 278 279 280 } 281 282 283 |
Was passiert bei Menüauswahl?
Aktionen.java |
---|
Das Hauptprogramm
Fenster.java |
---|
Letzte Änderung: 26.04.2012: 17:53:38 von X. Rendtel
Dieses Werk bzw. Inhalt steht unter einer Creative Commons Namensnennung-Weitergabe unter gleichen Bedingungen 3.0 Unported Lizenz.
Beruht auf einem Inhalt unter www.rendtel.de.